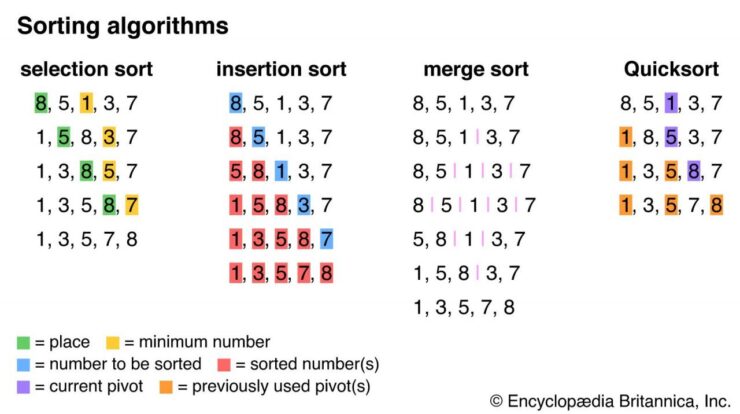
Sort definition, a fundamental concept in computer science, plays a pivotal role in organizing and structuring data for efficient processing. This guide delves into the intricacies of sorting, exploring its types, data structures, efficiency considerations, and real-world applications.
From basic algorithms like bubble sort to advanced techniques such as radix sort, we’ll uncover the nuances of sorting and its impact on data management and analysis.
Introduction
Sorting, the process of arranging data in a specific order, plays a crucial role in various domains. From organizing large datasets to optimizing search algorithms, sorting techniques are essential for efficient data processing.
Types of Sorting Algorithms
There are numerous sorting algorithms, each with its strengths and weaknesses. Some common types include:
- Bubble Sort:Compares adjacent elements and swaps them if they are out of order.
- Insertion Sort:Builds a sorted array by inserting each element into its correct position.
- Selection Sort:Finds the minimum element and swaps it with the first element, then repeats for the remaining elements.
- Merge Sort:Divides the array into smaller subarrays, sorts them, and merges them back together.
- Quick Sort:Uses a divide-and-conquer approach, selecting a pivot element and partitioning the array around it.
These algorithms vary in their time complexity and space requirements, making them suitable for different applications.
Data Structures for Sorting: Sort Definition
The choice of data structure can impact the performance of sorting algorithms. Common data structures used for sorting include:
- Arrays:Fixed-size collections of elements that can be accessed using an index.
- Linked Lists:Collections of nodes, each containing data and a reference to the next node.
- Trees:Hierarchical data structures that organize data in a parent-child relationship.
The selection of a data structure depends on factors such as the size of the data, the type of sorting algorithm used, and the desired performance characteristics.
Efficiency Considerations
The efficiency of sorting algorithms is influenced by factors such as:
- Input Size:The number of elements in the array.
- Data Distribution:The distribution of values within the array (e.g., sorted, partially sorted, or random).
- Sorting Algorithm Choice:The algorithm used to sort the array.
Understanding these factors and selecting an appropriate algorithm can significantly improve sorting performance.
Advanced Sorting Techniques
Beyond traditional sorting algorithms, there are specialized techniques that offer enhanced performance for certain applications:
- Radix Sort:Sorts elements by their individual digits or bits.
- Bucket Sort:Divides the input into smaller buckets and sorts each bucket independently.
- Heap Sort:Uses a heap data structure to build a sorted array.
These techniques have specific advantages and are used in applications that require high efficiency and scalability.
Real-World Applications of Sorting
Sorting is ubiquitous in various domains:
- Data Processing:Organizing large datasets for analysis and visualization.
- Machine Learning:Training models by organizing and pre-processing data.
- Database Management:Maintaining sorted indexes for efficient data retrieval.
- Web Search Engines:Ranking search results based on relevance and user preferences.
Sorting algorithms are essential tools for managing and processing data effectively in a wide range of applications.
Closure
In conclusion, sort definition is a cornerstone of data processing, enabling us to transform unsorted data into organized and meaningful information. By understanding the various sorting algorithms and their complexities, we can optimize data management and unlock the full potential of data-driven applications.
Top FAQs
What is the significance of sorting in data processing?
Sorting is crucial for organizing and retrieving data efficiently. It allows us to arrange data in a specific order, making it easier to search, filter, and analyze.
How do different data structures impact sorting performance?
The choice of data structure, such as arrays or linked lists, can significantly affect sorting efficiency. Arrays provide faster access and manipulation, while linked lists offer flexibility and dynamic memory allocation.